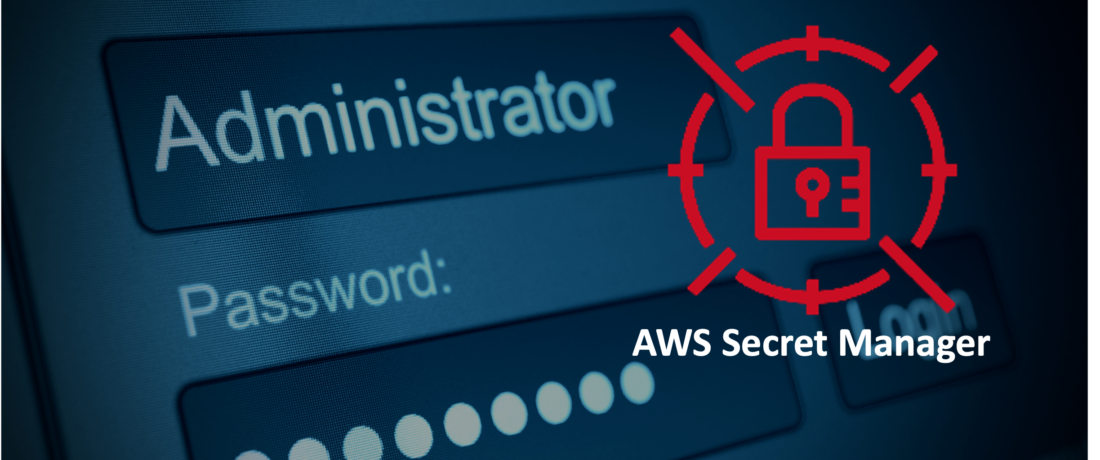
AWS Secrets Manager is a service to help you protect secrets needed to access your applications, services, and IT resources. The service enables you to easily rotate, manage, and retrieve database credentials, API keys, and other secrets throughout their lifecycle. Users and applications retrieve secrets with a call to Secrets Manager APIs, eliminating the need to hardcode sensitive information in plain text. Secrets Manager offers secret rotation with built-in integration for Amazon RDS, Amazon Redshift, and Amazon DocumentDB. Also, the service is extensible to other types of secrets, including API keys and OAuth tokens. In addition, Secrets Manager enables you to control access to secrets using fine-grained permissions and audit secret rotation centrally for resources in the AWS Cloud, third-party services, and on-premises.
This article provides a step by step guide on how to use the AWS Secrets Manager in a PHP program
Prerequisites:
High-level Steps
You can use the AWS Secrets manager console for creating the secrets.
Or if you’ve already signed in on AWS console, go to the Secrets Manager
To make requests to Amazon Web Services from a PHP program, you need to supply AWS access keys, also known as credentials.
You can do this in the following ways:
In this document, we’re going to use the first approach, i.e. default credential provider chain, and store the credential in a credential file.
To enable a user to call AWS API services, you must create an IAM policy for an IAM user, which controls access to the API Gateway entities, and then attach the policy to the IAM user. The following steps describe how to create your IAM policy.
To create your own IAM policy
You have just created an IAM policy. It won’t have any effect until you attach it to an IAM user, to an IAM group containing the user, or to an IAM role assumed by the user.
To create an IAM user for an application with attaching the created IAM policy.
In this section, you’re going to create an EC2 with LAMP. It will be used for developing your PHP page in the later section. LAMP is an open-source software stack that provides a framework for creating PHP-based high-performance websites and applications with ease.
In this section, you’re going to build a PHP program to get the secret values from the AWS Secrets Manager. You’ll need to login to the command console through SSH, install the required AWS SDK for PHP before you can start building your PHP program.
To SSH to your EC2.
To install the AWS SDK for PHP Version 3
You’re going to install AWS SDK for PHP as a dependency via Composer.
Composer is the recommended way to install the AWS SDK for PHP. Composer is a tool for PHP that manages and installs the dependencies of your project.
To prepare the PHP code to get secret values
In the final step, you’re going to register the AWS access key in a credential file for the PHP program to access the required AWS service.
A credentials file is a plaintext file that contains your access keys. The file must:
We use this method in the Get Secret Value code.
Using an AWS credentials file offers the following benefits:
To prepare the AWS credential